SnackBar
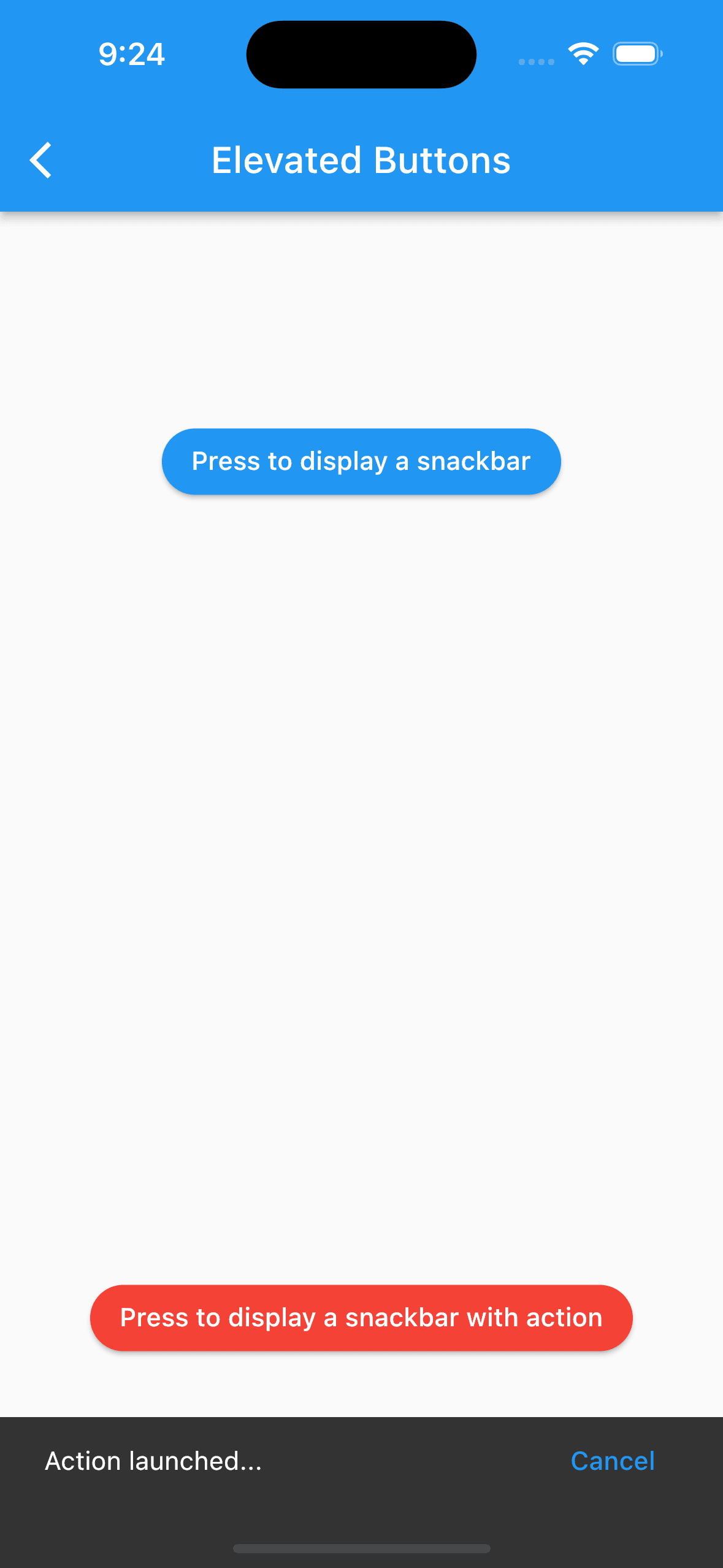
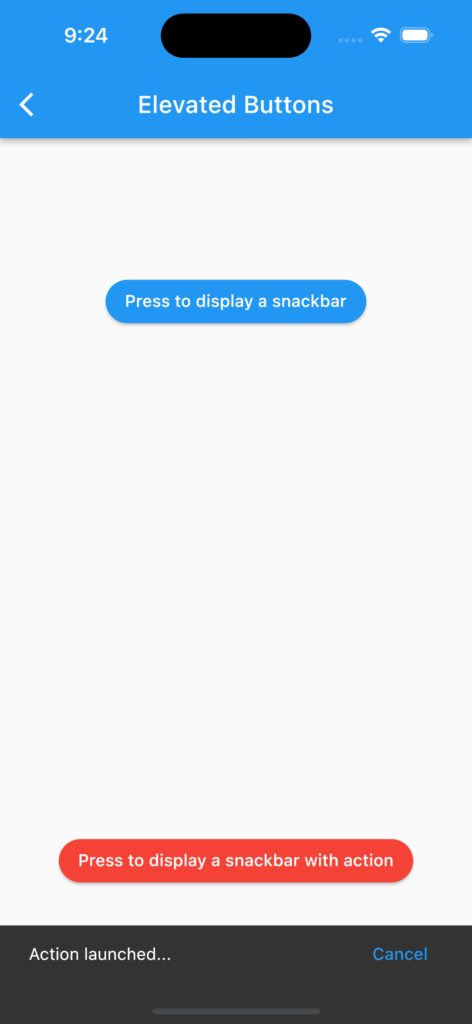
Shows 2 snackbars (small window popping up from the bottom of the screen): 1 simple snackbar displaying a message, and another snackbar displaying a message and an action button.
import 'package:flutter/material.dart';
class ShowSnackBar extends StatefulWidget {
const ShowSnackBar({Key? key}) : super(key: key);
@override
_ShowSnackBarState createState() => _ShowSnackBarState();
}
class _ShowSnackBarState extends State<ShowSnackBar> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text("Elevated Buttons")),
body: Container(
padding: const EdgeInsets.all(20),
alignment: Alignment.center,
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
buttonForSimpleSnackBar(context),
const SizedBox(height: 50),
buttonForSnackBarWithAction(context),
],
)
)
);
}
/// **********************************************************************
/// Button for simple snackbar example
/// **********************************************************************
Widget buttonForSimpleSnackBar(BuildContext context) {
return ElevatedButton(
onPressed: () {
displaySnackBar(context);
},
style: ElevatedButton.styleFrom(
backgroundColor: Colors.blue,
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(20.0))
),
child: const Text("Press to display a snackbar")
);
}
/// **********************************************************************
/// Simple snackbar
/// **********************************************************************
void displaySnackBar(BuildContext context) {
var snackBar = const SnackBar(content: Text("Simple snackbar"));
ScaffoldMessenger.of(context).showSnackBar(snackBar);
}
/// **********************************************************************
/// Button for snackbar with action example
/// **********************************************************************
Widget buttonForSnackBarWithAction(BuildContext context) {
return ElevatedButton(
onPressed: () {
displaySnackBarAction(context);
},
style: ElevatedButton.styleFrom(
backgroundColor: Colors.red,
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(20.0))
),
child: const Text("Press to display a snackbar with action")
);
}
/// **********************************************************************
/// Snackbar with action example
/// **********************************************************************
void displaySnackBarAction(BuildContext context) {
var snackBar = SnackBar(
content: const Text("Action launched..."),
action: SnackBarAction(
label: "Cancel",
onPressed: () {
print("Action canceled"); },
),
);
ScaffoldMessenger.of(context).showSnackBar(snackBar);
}
}