Floating Action Button
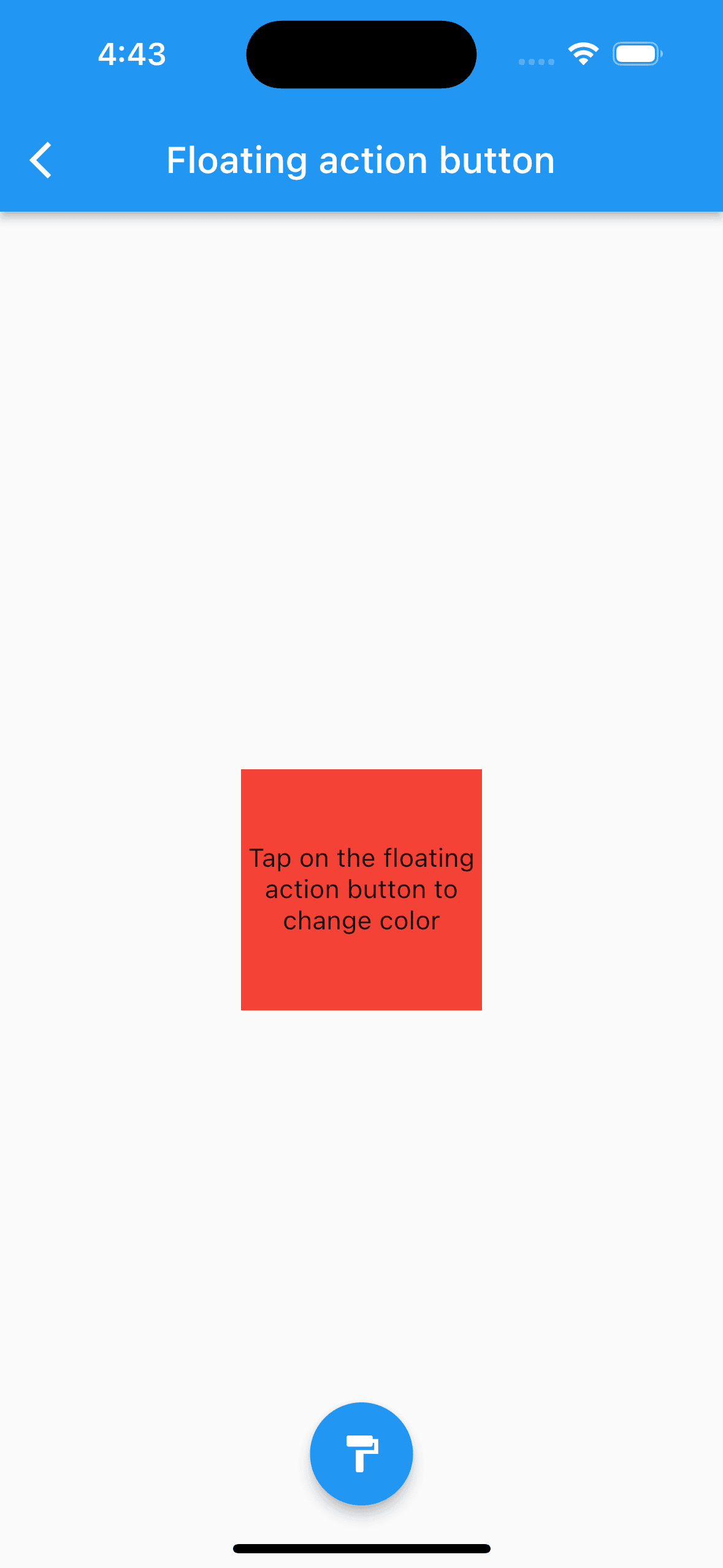
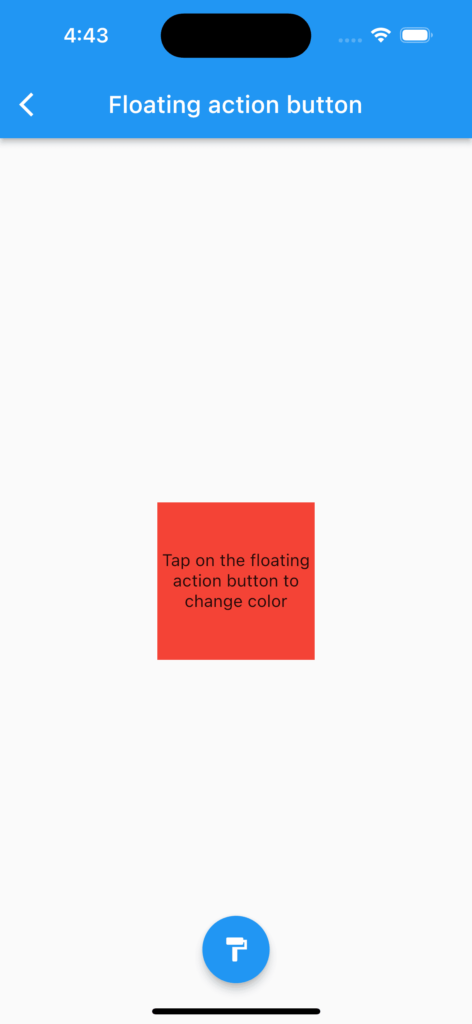
Demonstrating 1 single floating action button to toggle the color of the center box. The floating action button is a property of the Scaffold class, its location (here .centerDocked) is also a property of the Scaffold class. Check out the Scaffold class description for available options.
import 'package:flutter/material.dart';
class ShowFloatingActionButton extends StatefulWidget {
const ShowFloatingActionButton({Key? key}) : super(key: key);
@override
_ShowFloatingActionButtonState createState() => _ShowFloatingActionButtonState();
}
class _ShowFloatingActionButtonState extends State<ShowFloatingActionButton> {
var _toggleColor = false;
@override
Widget build(BuildContext context) {
double width = MediaQuery.of(context).size.width;
double height = MediaQuery.of(context).size.height;
return Scaffold(
appBar: AppBar(title: const Text("Floating action button"),),
body: Container(
padding: const EdgeInsets.all(20),
alignment: Alignment.center,
child: Center(
child: Container(
alignment: Alignment.center,
width: width/3,
height: width/3,
color:
(_toggleColor)
? Colors.green
: Colors.red,
child: const Text("Tap on the floating action button to change color",
textAlign: TextAlign.center,)
),
),
),
floatingActionButton: displayFloatingActionButton(context),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
);
}
Widget displayFloatingActionButton(BuildContext context) {
return FloatingActionButton(
child: const Icon(Icons.format_paint),
onPressed: () {
setState(() {
_toggleColor = !_toggleColor;
});
});
}
}